能力值:
(RANK:730 )
|
-
-
2 楼
这很有意思啊!
|
能力值:
( LV12,RANK:290 )
|
-
-
3 楼
有毒
这很有意思啊!
居然得到毒哥的认可了!
|
能力值:
(RANK:730 )
|
-
-
4 楼
pureGavin
居然得到毒哥的认可了!
别骂了别骂了,这个内容我真的不熟,就觉得很好玩
|
能力值:
( LV15,RANK:880 )
|
-
-
5 楼
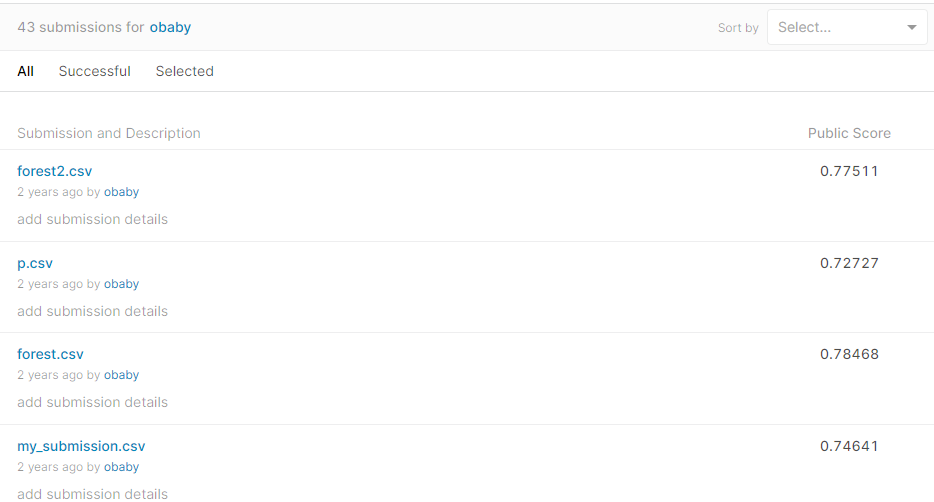
这个我也做过,尝试了不同的方法。不过效果吗,比大神的差远了
|
能力值:
( LV3,RANK:20 )
|
-
-
6 楼
喜欢这种主题文章
|
能力值:
( LV12,RANK:290 )
|
-
-
7 楼
obaby
这个我也做过,尝试了不同的方法。不过效果吗,比大神的差远了
怎么也不贴一下你的代码,我感觉分数之所以提不上去,就是因为有些与幸存率无关的数据比如passengerID也被当做特征算进去了,随机森林对这种有无关特征的样本就容易过拟合
|
能力值:
( LV15,RANK:880 )
|
-
-
8 楼
pureGavin
怎么也不贴一下你的代码,我感觉分数之所以提不上去,就是因为有些与幸存率无关的数据比如passengerID也被当做特征算进去了,随机森林对这种有无关特征的样本就容易过拟合
是的,数据清洗和特征选择挺关键的。给的数据本身纬度不少但是缺失数据较多,缺失数据的补充也是个技术活。http://h4ck.org.cn/2019/11/%E5%9F%BA%E4%BA%8Erandomforestclassifier%E7%9A%84titanic%E7%94%9F%E5%AD%98%E6%A6%82%E7%8E%87%E5%88%86%E6%9E%90/ 代码现在手上没有,直接提个我的blog链接吧
|
能力值:
( LV12,RANK:290 )
|
-
-
9 楼
obaby
是的,数据清洗和特征选择挺关键的。给的数据本身纬度不少但是缺失数据较多,缺失数据的补充也是个技术活。http://h4ck.org.cn/2019/11/%E5%9F%BA%E4%BA%8Erando ...
大佬牛逼,带带我可以吗
|
能力值:
( LV15,RANK:880 )
|
-
-
10 楼
pureGavin
大佬牛逼,带带我可以吗 大佬,表酱紫~~我也是菜到起飞啊,业余爱好干这个,与实际干这个的水平差的太多了。可不敢害人啊~~
最后于 2022-1-7 10:31
被obaby编辑
,原因:
|
能力值:
( LV15,RANK:910 )
|
-
-
11 楼
为啥不用Pytorch或者tf
|
能力值:
( LV12,RANK:290 )
|
-
-
12 楼
1900
为啥不用Pytorch或者tf
杀鸡焉用牛刀
|
能力值:
( LV2,RANK:10 )
|
-
-
13 楼
我一直想问下,这是一个中专生的水平?兄弟,方便问下你多大了吗
|
能力值:
( LV12,RANK:290 )
|
-
-
14 楼
月明呀
我一直想问下,这是一个中专生的水平?兄弟,方便问下你多大了吗
22,有什么问题么?
|
能力值:
( LV2,RANK:10 )
|
-
-
15 楼
有自己代码 有例子代码 挺好的 期待后续更多
|
能力值:
( LV12,RANK:290 )
|
-
-
16 楼
ugui
有自己代码 有例子代码 挺好的 期待后续更多
后续不一定能发出来了,一直在搞得北京大学的一个竞赛,自动驾驶的目标识别,感觉问题有点儿大,有部分函数的运算过程看不懂…… 然后现在只能恶补数学知识了
|
|
|