https://github.com/acbocai/jeb_script
.
.
查询DEX中方法的交叉引用信息,
使用ActionXrefsData和ActionContext
.
.
查询一个函数被谁调用了,或查询它内部调用了谁,
关注:
INativeCodeAnalyzer
INativeCodeModel
ICallGraphManager
ICallGraph
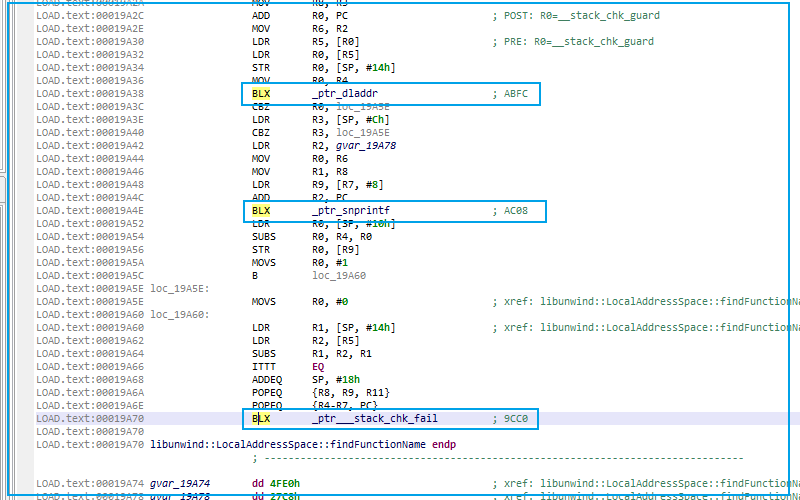
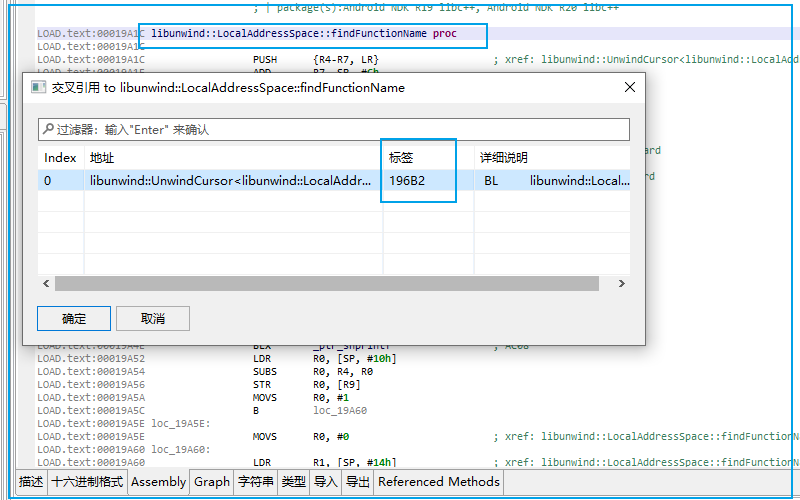
.
查询一个Function的交叉引用情况
或查询一个Block入口指令的引用交叉引用情况
关注:
INativeCodeAnalyzer
INativeCodeModel
IReferenceManager
IReference
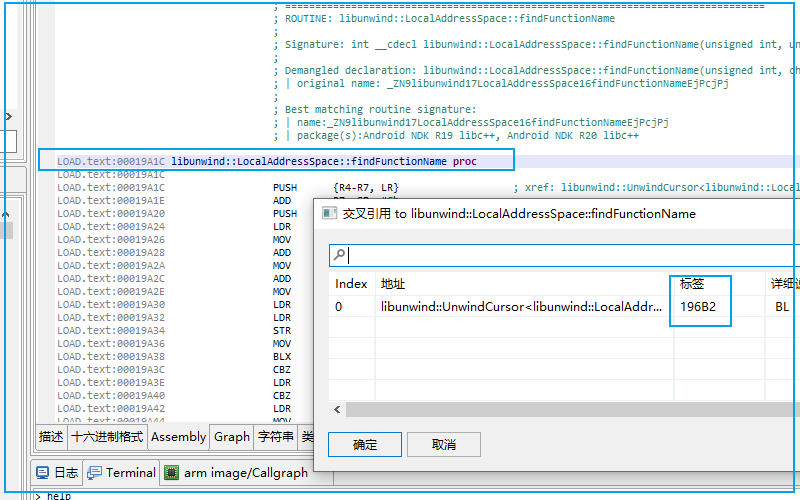
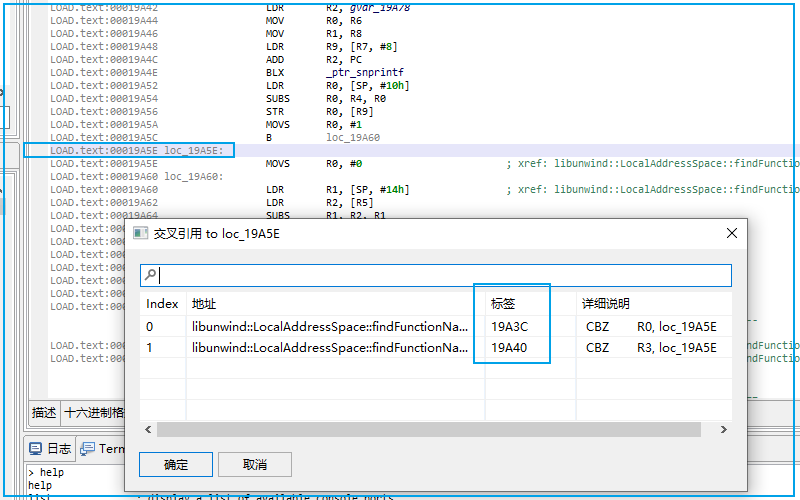
.
.
获取一条指令的地址,所属Function或Block.
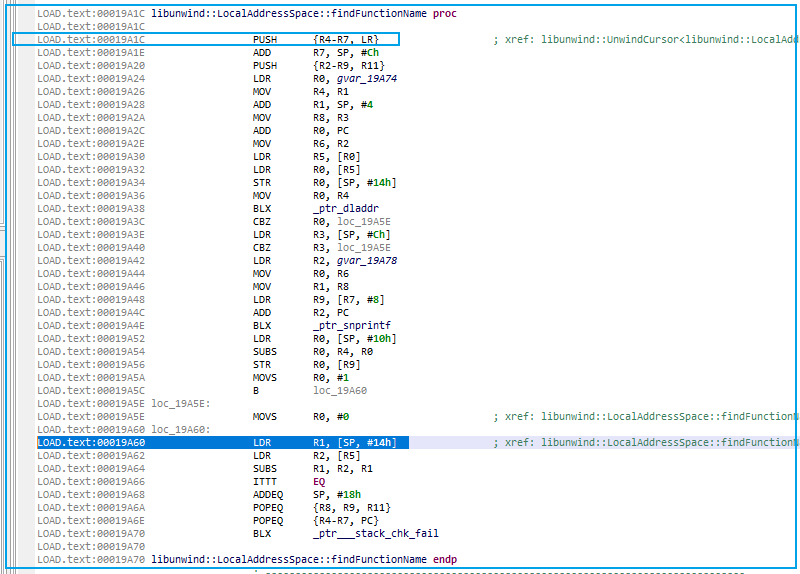
from
com.pnfsoftware.jeb.client.api
import
IClientContext
from
com.pnfsoftware.jeb.core
import
IRuntimeProject
from
com.pnfsoftware.jeb.core.actions
import
ActionXrefsData, Actions, ActionContext
from
com.pnfsoftware.jeb.core.units
import
IUnit
from
com.pnfsoftware.jeb.core.units.code.android
import
IDexUnit
from
com.pnfsoftware.jeb.core.units.code.android.dex
import
IDexMethod, IDexClass
def
Test(ctx):
input_path
=
r
"D:\tmp\2\project\about_dex_diff\code\jsq\jsq.dex"
class_sign
=
"Lcom/BestCalculatorCN/MyCalculator;"
method_sign
=
"Lcom/BestCalculatorCN/MyCalculator;->b(Lcom/BestCalculatorCN/MyCalculator;Ljava/lang/String;)V"
unit
=
ctx.
open
(input_path);
prj
=
ctx.getMainProject();
dexUnit
=
prj.findUnit(IDexUnit);
clz
=
dexUnit.getClass(class_sign);
method
=
dexUnit.getMethod(method_sign);
print
"------------------------------------------------"
actionXrefsData
=
ActionXrefsData()
actionContext
=
ActionContext(dexUnit, Actions.QUERY_XREFS, method.getItemId(),
None
)
if
unit.prepareExecution(actionContext,actionXrefsData):
for
xref_addr
in
actionXrefsData.getAddresses():
print
xref_addr
print
"------------------------------------------------"
actionXrefsData
=
ActionXrefsData()
actionContext
=
ActionContext(dexUnit, Actions.QUERY_XREFS, clz.getItemId(),
None
)
if
unit.prepareExecution(actionContext,actionXrefsData):
for
idx,xref_addr
in
enumerate
(actionXrefsData.getAddresses()):
print
idx,xref_addr
from
com.pnfsoftware.jeb.client.api
import
IClientContext
from
com.pnfsoftware.jeb.core
import
IRuntimeProject
from
com.pnfsoftware.jeb.core.actions
import
ActionXrefsData, Actions, ActionContext
from
com.pnfsoftware.jeb.core.units
import
IUnit
from
com.pnfsoftware.jeb.core.units.code.android
import
IDexUnit
from
com.pnfsoftware.jeb.core.units.code.android.dex
import
IDexMethod, IDexClass
def
Test(ctx):
input_path
=
r
"D:\tmp\2\project\about_dex_diff\code\jsq\jsq.dex"
class_sign
=
"Lcom/BestCalculatorCN/MyCalculator;"
method_sign
=
"Lcom/BestCalculatorCN/MyCalculator;->b(Lcom/BestCalculatorCN/MyCalculator;Ljava/lang/String;)V"
unit
=
ctx.
open
(input_path);
prj
=
ctx.getMainProject();
dexUnit
=
prj.findUnit(IDexUnit);
clz
=
dexUnit.getClass(class_sign);
method
=
dexUnit.getMethod(method_sign);
print
"------------------------------------------------"
actionXrefsData
=
ActionXrefsData()
actionContext
=
ActionContext(dexUnit, Actions.QUERY_XREFS, method.getItemId(),
None
)
if
unit.prepareExecution(actionContext,actionXrefsData):
for
xref_addr
in
actionXrefsData.getAddresses():
print
xref_addr
print
"------------------------------------------------"
actionXrefsData
=
ActionXrefsData()
actionContext
=
ActionContext(dexUnit, Actions.QUERY_XREFS, clz.getItemId(),
None
)
if
unit.prepareExecution(actionContext,actionXrefsData):
for
idx,xref_addr
in
enumerate
(actionXrefsData.getAddresses()):
print
idx,xref_addr
from
com.pnfsoftware.jeb.client.api
import
IClientContext
from
com.pnfsoftware.jeb.core
import
IRuntimeProject
from
com.pnfsoftware.jeb.core.units
import
IUnit, INativeCodeUnit
from
com.pnfsoftware.jeb.core.units.code.asm.analyzer
import
INativeCodeAnalyzer, INativeCodeModel, IReferenceManager, ICallGraphManager, ICallGraph, CallGraphVertex
from
com.pnfsoftware.jeb.core.units.code.asm.items
import
INativeMethodItem
def
Test(ctx):
assert
isinstance
(ctx,IClientContext)
input_path
=
r
"D:\tmp\2\project\about_dex_diff\code\xmly\libFace3D.so"
unit
=
ctx.
open
(input_path)
prj
=
ctx.getMainProject()
nativeCodeUnit
=
prj.findUnit(INativeCodeUnit)
bool
=
nativeCodeUnit.process()
nativeCodeAnalyzer
=
nativeCodeUnit.getCodeAnalyzer()
nativeCodeAnalyzer.analyze()
nativeCodeModel
=
nativeCodeAnalyzer.getModel()
callGraph
=
nativeCodeModel.getCallGraphManager().getGlobalCallGraph()
funcName
=
"libunwind::LocalAddressSpace::findFunctionName"
nativeMethodItem
=
nativeCodeUnit.getMethod(funcName)
print
">>> funcAddr:"
,
hex
(nativeMethodItem.getRoutineAddress())
callGraphVertexList
=
callGraph.getCallees(nativeMethodItem,
False
)
for
callGraphVertex
in
callGraphVertexList:
print
">>> Callee:"
,
hex
(callGraphVertex.getInternalAddress().getAddress())
callerList
=
callGraph.getCallers(nativeMethodItem,
False
)
for
caller
in
callerList:
print
">>> Callers:"
,
hex
(caller)
from
com.pnfsoftware.jeb.client.api
import
IClientContext
from
com.pnfsoftware.jeb.core
import
IRuntimeProject
from
com.pnfsoftware.jeb.core.units
import
IUnit, INativeCodeUnit
from
com.pnfsoftware.jeb.core.units.code.asm.analyzer
import
INativeCodeAnalyzer, INativeCodeModel, IReferenceManager, ICallGraphManager, ICallGraph, CallGraphVertex
from
com.pnfsoftware.jeb.core.units.code.asm.items
import
INativeMethodItem
def
Test(ctx):
assert
isinstance
(ctx,IClientContext)
input_path
=
r
"D:\tmp\2\project\about_dex_diff\code\xmly\libFace3D.so"
unit
=
ctx.
open
(input_path)
prj
=
ctx.getMainProject()
nativeCodeUnit
=
prj.findUnit(INativeCodeUnit)
bool
=
nativeCodeUnit.process()
nativeCodeAnalyzer
=
nativeCodeUnit.getCodeAnalyzer()
nativeCodeAnalyzer.analyze()
nativeCodeModel
=
nativeCodeAnalyzer.getModel()
callGraph
=
nativeCodeModel.getCallGraphManager().getGlobalCallGraph()
funcName
=
"libunwind::LocalAddressSpace::findFunctionName"
nativeMethodItem
=
nativeCodeUnit.getMethod(funcName)
print
">>> funcAddr:"
,
hex
(nativeMethodItem.getRoutineAddress())
callGraphVertexList
=
callGraph.getCallees(nativeMethodItem,
False
)
for
callGraphVertex
in
callGraphVertexList:
print
">>> Callee:"
,
hex
(callGraphVertex.getInternalAddress().getAddress())
callerList
=
callGraph.getCallers(nativeMethodItem,
False
)
for
caller
in
callerList:
print
">>> Callers:"
,
hex
(caller)
[招生]科锐逆向工程师培训(2024年11月15日实地,远程教学同时开班, 第51期)
最后于 2020-10-28 14:32
被爱吃菠菜编辑
,原因: