能力值:
( LV12,RANK:530 )
|
-
-
2 楼
 谢谢分享~~
|
能力值:
( LV3,RANK:20 )
|
-
-
3 楼
谢谢分享…学习
|
能力值:
( LV11,RANK:180 )
|
-
-
4 楼
猛男~~
|
能力值:
( LV2,RANK:10 )
|
-
-
5 楼
不错的吖,怎么没人顶呢/
|
能力值:
( LV4,RANK:40 )
|
-
-
6 楼
nice,原来云查杀是这样一个原理
|
能力值:
( LV12,RANK:210 )
|
-
-
7 楼
云查杀依据庞大的黑白名单库+N种反病毒的软件扫描+人工分析
1、黑白名单(关键就是及时、全面)
2、N种反病毒查杀(速度)
3、人工分析(都没扫出来的,人再去看一看,不确定有这一环节)
4、杀掉发现的病毒(杀完病毒,系统可否正常使用)
|
能力值:
( LV12,RANK:210 )
|
-
-
8 楼
云查杀在互联网时代效果还是蛮好的吧。
|
能力值:
( LV2,RANK:10 )
|
-
-
9 楼
思路很好这个 代码
|
能力值:
( LV2,RANK:10 )
|
-
-
10 楼
这个 前年 就玩过了
|
能力值:
( LV2,RANK:10 )
|
-
-
11 楼
顶贴支持 ~~
|
能力值:
( LV2,RANK:10 )
|
-
-
12 楼
期待更新这个软件 不错
|
能力值:
( LV2,RANK:10 )
|
-
-
13 楼
估计国内那些云也是如此
|
能力值:
( LV4,RANK:50 )
|
-
-
14 楼
|
能力值:
( LV12,RANK:210 )
|
-
-
15 楼
单机用户。。。。亿级试试
|
能力值:
( LV2,RANK:10 )
|
-
-
16 楼

思路那是相当的棒
|
能力值:
( LV2,RANK:10 )
|
-
-
17 楼
很强悍,收藏了,研究下
|
能力值:
( LV2,RANK:10 )
|
-
-
18 楼
谢谢楼主,思路不错呀
|
能力值:
( LV2,RANK:10 )
|
-
-
19 楼
[QUOTE=hawkish;946697]思路很好,花了点时间,用MFC重写了。。。。
KillVirus.rar
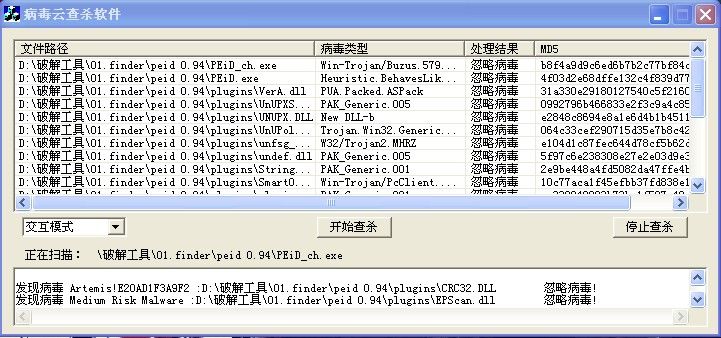 [/QUOTE]
嘿嘿…不错…看看
|
能力值:
( LV4,RANK:40 )
|
-
-
20 楼
感谢LZ
不过如果只是MD5的话 多加一个字节就可以洗洗睡了吧?
|
能力值:
( LV2,RANK:10 )
|
-
-
21 楼
流量很贵的,哈哈,云端表示都这么玩,情何以堪
|
能力值:
( LV4,RANK:50 )
|
-
-
22 楼
思路很好~~~支持一下~
|
能力值:
( LV2,RANK:10 )
|
-
-
23 楼
同感,这个贵在思路清晰
|
能力值:
( LV2,RANK:10 )
|
-
-
24 楼
国内云查杀估计都是这样么?
|
能力值:
( LV2,RANK:10 )
|
-
-
25 楼
總感覺這不是雲查殺吧...........
|
|
|